Git Stash: Tutorial & Tips (2023)
A tutorial explaining the process of creating, applying, and managing stashes using the 'git stash' command.
TL;DR
Use git stash
to save changes without committing them before switching to a different branch
Written by @bazamel_
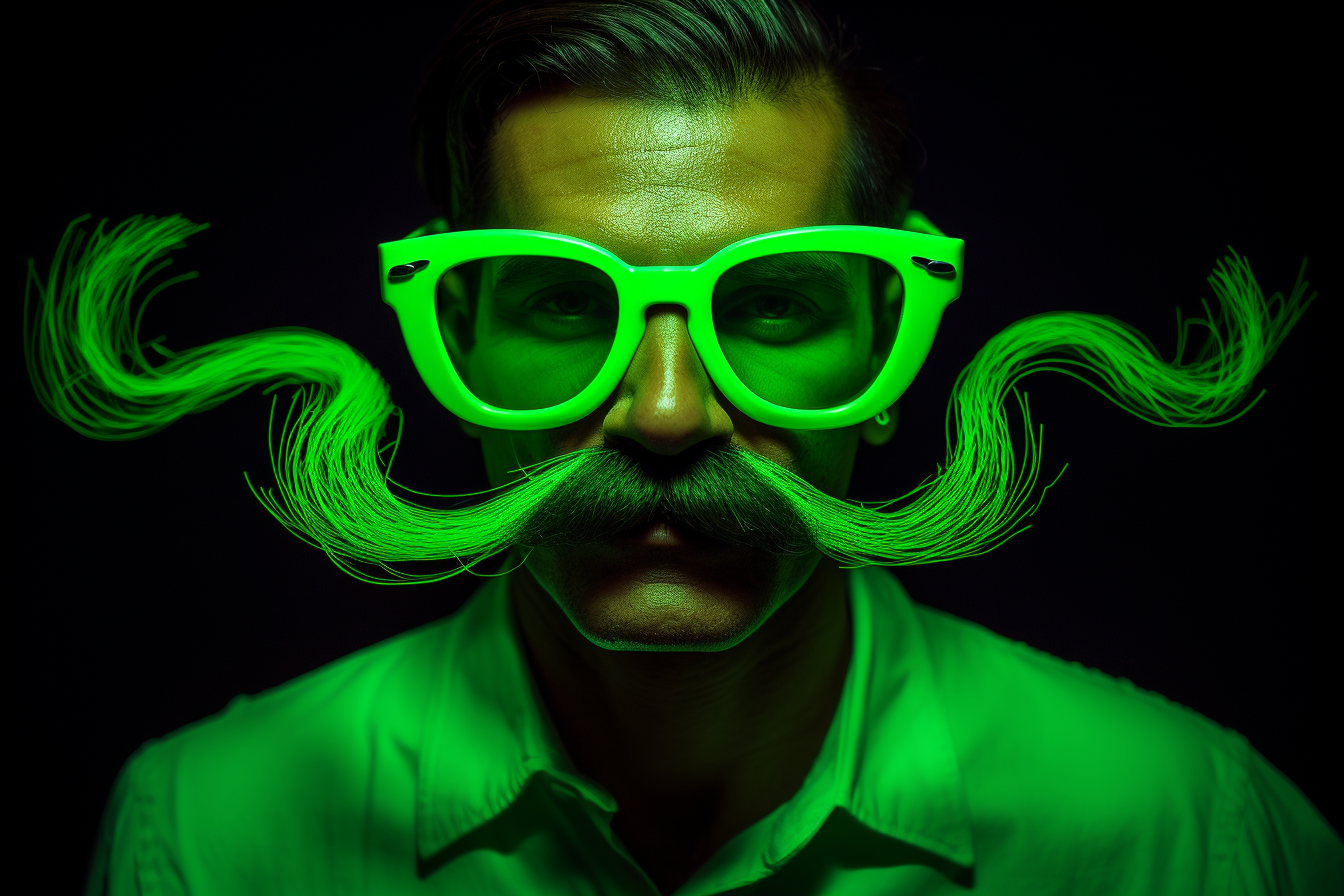
The command “git stash” temporarily saves the current changes in a separate location, allowing you to switch to a different branch without committing the changes.
How do I use it?
Using the git stash
command
Sometimes, when working on a Git project, you may find yourself in a situation where you need to switch to a different branch or task, but you’re not ready to commit your current changes. In such cases, the git stash
command can prove to be very useful.
How git stash
works
The git stash
command allows you to save your changes in a temporary storage area called the stash. It’s like creating a checkpoint of your work that you can come back to later.
When you run git stash
, Git will take all your uncommitted changes, including those staged for commit, and revert your working directory to the last commit. It also saves the changes in the stash.
Once your working directory is clean, you can switch to another branch or task without any conflicts. When you’re ready to go back to your previous work, you can retrieve the changes from the stash.
Example usage
Here’s a step-by-step example of how to use the git stash
command:
- Make changes to your code or files in the working directory.
- Stage the files you want to commit using
git add
. - Run
git stash
:
$ git stash
- Git will save your changes in the stash and revert your working directory to the last commit.
- Switch to a different branch or work on a different task.
- When you’re ready to continue your previous work, switch back to your original branch or task.
- Retrieve the changes from the stash using
git stash apply
:
$ git stash apply
- Git will apply the stashed changes to your working directory, allowing you to continue where you left off.
Remember that git stash
is not a replacement for commits. It’s a temporary solution to save your changes while working on different branches or tasks without interfering with your progress.
Why is it useful?
-
Temporary Code Switch - When you need to switch to a different branch to work on a different feature or fix a bug, but your current branch has uncommitted changes -
git stash
allows you to save your changes so you can switch branches without losing your work. -
Code Experimentation - When you want to experiment with different code changes, but you’re not sure if they will work or you might want to revert them later -
git stash
allows you to save your changes, revert back to the original state, and easily apply the experiment changes later on. -
Interrupted Development - When you are in the middle of working on a specific feature or fixing a bug, but an urgent task unrelated to your current work arises -
git stash
allows you to save your changes, switch to the urgent task, and then come back to your previous work later. -
Branch Initialization - When you need to create a new branch but don’t want to carry forward any uncommitted changes from the current branch -
git stash
allows you to stash the changes, create the new branch, and then apply the stashed changes seamlessly. -
Conflict Resolution - When you encounter merge conflicts during a
git pull
orgit merge
operation, and you want to switch to another branch to fix the conflicts, but don’t want to lose the current changes -git stash
lets you stash the current changes, switch branches, resolve the conflicts, and continue working with the original changes later on. -
Accidental Changes - When you mistakenly make changes to files that should not have been modified -
git stash
allows you to stash the erroneous changes and revert the files to their original state.
Remember, git stash
is a powerful tool to temporarily save your changes, switch branches or fix conflicts, and then reapply the saved changes when needed - making it an invaluable command in your Git workflow.
Interesting options
Git Stash
Git stash allows you to save changes in a temporary area so that you can switch branches or work on something else without committing those changes.
Options:
-
git stash save <message> - Saves changes with a descriptive message.
git stash save "WIP: Work in progress"
-
git stash apply - Applies the most recent stash without removing it from the stash list.
git stash apply
-
git stash pop - Applies the most recent stash and removes it from the stash list.
git stash pop
-
git stash list - Lists all stashes with a unique identifier and description.
git stash list
-
git stash show <stash> - Shows the changes in the specified stash.
git stash show stash@{2}
-
git stash drop <stash> - Removes the specified stash from the stash list.
git stash drop stash@{1}
-
git stash clear - Removes all stashed changes.
git stash clear
-
git stash branch <branch-name> - Creates a new branch from a stash.
git stash branch new-feature stash@{0}
-
git stash create - Creates a new stash without applying it.
git stash create
-
git stash store - Stores a given stash in a new stash entry without modifying the working tree or the index.
git stash store -m "WIP: Feature in progress"
The git stash
command offers various options to stash and manage changes in your Git repository.
Best practices
Best Practices for Using git stash
When using git stash
in your development workflow, keep the following best practices in mind:
- Commit your changes before stashing - It ensures that you have a clean working directory, making it easier to apply and manage stashes later.
- Give a descriptive and meaningful stash message - Clear stash messages make it easier to identify and apply the correct stash.
- Use
git stash push
instead ofgit stash save
- Thepush
command provides greater flexibility and clarity in specifying changes to be stashed. - Consider using the
--patch
option - It allows you to selectively stash specific changes from a file, keeping your stash focused and relevant. - Use
git stash branch
to create a new branch for a stash - This helps to separate the changes from the stash and work on them without affecting your current branch. - Use
git stash apply
followed bygit stash drop
instead ofgit stash pop
- It allows you to apply the stash and keep it in the stash list for future use. - Review and clean up your stash list regularly - It helps to keep track of your stashes and avoid cluttering your stash list with unnecessary items.
- Combine multiple stashes into one if they are related - Consolidating related stashes simplifies management and makes it easier to apply them collectively if needed.
- Avoid using
git stash
as a replacement for commit - Stashing is primarily meant for temporary purposes, so it’s essential to commit your changes when appropriate. - Ensure that your working directory is clean before applying a stash - Having a clean working directory minimizes conflicts when applying stashed changes.
Remember, effective usage of git stash
can streamline your development process, allowing you to work on separate tasks without the need for multiple branches.